connect node in javascript with php and database
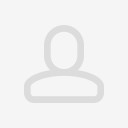
<!doctytml>
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.5.7/angular.min.js"></script>
</head>
<style>
body {
background:url("fd.jpg");
font-family: 'Titillium Web', sans-serif;
background-size:cover;
width:100%;
height:100%
}
#control_panel {
size:20px;
float: left;
width: 7%;
height: 7px;
background-color: #3e2217;
}
#divider {
float: left;
width: 10%;
height: 00px;
}
#control_panel button {
background-color: #4a5662;
color:#a9bbc5;;
font-size: 20px;
width: 100%;
}
#control_panel button:hover {
cursor: pointer;
}
#information_panel {
font-color:#c7957a;
;
box-shadow: 0px 0px 40px 16px rgba(18,18,18,1.00);
}
#information_panel ul {
background-color:#4a5662;
list-style: none;
padding: 3px;
}
.section {
background-color:#a9bbc5;;
color: white;
font-size: 20px;
text-align: center;
padding-bottom: 2px;
padding-top: 2px;
}
#header {
text-align: center;
box-shadow: 0px 0px 40px 16px rgba(18,18,18,1.00);
font-size: 24px;
}
#main {
float: left;
width: %;
height: 800px;
}
#main canvas {
}
#main input {
width: 100px;
height: 50px;
}
</style>
<body onload="initialize()" style="
width: 2000px;
overflow-x: scroll;">
<?php
$con = mysqli_connect('localhost', 'root', '', 'courses');
if (mysqli_connect_errno())
{
var_dump("Failed to connect to MySQL: " . mysqli_connect_error());
exit;
}
$query = 'SELECT * FROM courses';
$result = mysqli_query($con, $query);
$array = array();
while ($row = mysqli_fetch_assoc($result)) {
$array[] = ['id' => $row['id'], 'name' => $row['name'], 'parent' => $row['parent_id']];
}
$JsonArray = json_encode($array);
?>
<div id="header">
computer information System
</div>
<div id="control_panel">
<div class="section">
Control Panel
</div>
<button id="add_child">
Add Child
</button>
<button id="remove_node">
Remove Nod
</button>
<button id="zoom_in">
Zoom In
</button>
<button id="zoom_out">
Zoom Out
</button>
<button id="Go">
Go
</button>
<div class="section">
Information Panel
</div>
<div id="information_panel">
</div>
</div>
<div id="divider">
</div>
<div id="main">
<canvas id="canvas" width="1777" height="1000" style="cursor: auto;"></canvas></div>
<script>//TREE.js is a library for creating and drawing properly displayed family trees on the HTML canvas element.
//This is a work in progress.
//Authors: Andy MacDonald
//16 June 2015
//--------------------------------------------------------------------------------------------------------
var TREE = (function () {
"use strict";
var uID = 0,
config = {
width: 70,
height: 70,
color: "black",
bgcolor: "#a9bbc5"
},
Tree = function (text, parentId, width, height, color, bgcolor, treeData) {
this.uid = uID += 1;
this.parentId = parentId || -1;
this.text = text;
this.width = width || config.width;
this.height = height || config.height;
this.color = color || config.color;
this.bgcolor = bgcolor || config.bgcolor;
this.treeData = treeData || {};
this.xPos = 0;
this.yPos = 0;
this.prelim = 0;
this.modifier = 0;
this.leftNeighbor = null;
this.rightNeighbor = null;
this.parentTree = null;
this.children = [];
};
/**
* Gets the vertical level of the tree.
* @returns {*} A number representing the vertical level of the tree.
*/
Tree.prototype.getLevel = function () {
return this.parentId === -1 ? 0 : this.parentTree.getLevel() + 1;
};
/**
* Sets the text color of the tree node.
* @param color The color to change it to.
*/
Tree.prototype.setColor = function (color) {
this.color = color;
};
/**
* Sets the background color of the tree node.
* @param color The color to change it to.
*/
Tree.prototype.setbgColor = function (color) {
this.bgcolor = color;
};
/**
* Visually changes the style of the node if it is 'selected'.
* @param bool A true or false value representing if node is selected or not.
*/
Tree.prototype.selected = function (bool) {
if (bool) {
this.setColor("#a9bbc5");
this.setbgColor("#4a5662");
} else {
this.setColor("##4a5662");
this.setbgColor("#a9bbc5");
}
};
/**
* Returns the number of children of this tree.
* @returns {Number} The number of children.
*/
Tree.prototype.numChildren = function () {
return this.children.length;
};
/**
* Returns the left sibling of this tree.
* @returns {*} The left sibling or null.
*/
Tree.prototype.getLeftSibling = function () {
return this.leftNeighbor && this.leftNeighbor.parentTree === this.parentTree ? this.leftNeighbor : null;
};
/**
* Returns the right sibling of this tree.
* @returns {*} The right sibling tree or null.
*/
Tree.prototype.getRightSibling = function () {
return this.rightNeighbor && this.rightNeighbor.parentTree === this.parentTree ? this.rightNeighbor : null;
};
/**
* Returns the child at a specified index.
* @param index The specified index.
* @returns {*} The child if found or null.
*/
Tree.prototype.getChildAt = function (index) {
return this.children[index];
};
/**
* Searches children and returns a tree by UID.
* @param id The UID to search for.
* @returns {*} The child if found or null.
*/
Tree.prototype.getChild = function (id) {
var i;
for (i = 0; i < this.children.length; i++) {
if (this.children[i].uid === id) {
return this.children[i];
}
}
};
/**
* Returns an X value representing the center location of all this tree's children.
* @returns {*} The cent0er X value.
*/
Tree.prototype.getChildrenCenter = function () {
var firstChild = this.getFirstChild(),
lastChild = this.getLastChild();
return firstChild.prelim + (lastChild.prelim - firstChild.prelim + lastChild.width) / 2;
};
/**
* Return the first child of this tree.
* @returns {Object} The child node.
*/
Tree.prototype.getFirstChild = function () {
return this.getChildAt(0);
};
/**
* Gets the last child of this tree.
* @returns {Object} The child node.
*/
Tree.prototype.getLastChild = function () {
return this.getChildAt(this.numChildren() - 1);
};
/**
* Adds a tree node to the children to this tree.
* @param tree The tree to be added.
*/
Tree.prototype.addChild = function (tree) {
tree.parentTree = this;
tree.parentId = this.uid;
this.children.push(tree);
};
/**
* Find and return a descendant by the UID.
* @param id The UID to search for.
* @returns {*} The found tree node or null if not found.
*/
Tree.prototype.getDescendent = function (id) {
var children = this.children;
var found;
if (this.getChild(id)) {
return this.getChild(id);
}
else {
for (var i = 0; i < children.length; i++) {
found = children[i].getDescendent(id);
if (found) {
return found;
}
}
}
};
return {
config : config,
/**
* Create and return a new tree.
* @constructor
* @param text The textual representation of the tree.
* @returns {Tree} The newly created tree.
*/
create: function (text) {
return new Tree(text);
},
/**
* Removes a tree from it's parents list of children. This effectively removes the tree and all of its
* descendants from an existing tree.
* @param tree The tree to be removed.
*/
destroy: function (tree) {
if (!tree.parentTree) {
alert("Removing root node not supported at this time");
return;
}
var children = tree.parentTree.children;
for (var i = 0; i < children.length; i++) {
if (children[i].uid === tree.uid) {
children.splice(i, 1);
break;
}
}
},
/**
* Get an array of all nodes in a tree.
* @param tree The tree.
* @returns {Array} An array of tree nodes.
*/
getNodeList: function (tree) {
var nodeList = [];
nodeList.push(tree);
for (var i = 0; i < tree.numChildren(); i++) {
nodeList = nodeList.concat(this.getNodeList(tree.getChildAt(i)));
}
return nodeList;
},
/**
* Clears the canvas.
* @param context The 2-d context of an html canvas element.
*/
clear: function (context) {
context.clearRect(0, 0, context.canvas.width, context.canvas.height);
},
/**
* Draws a well-formed tree on a canvas.
* @param context The 2-d context of a canvas html element.
* @param tree The tree that will be drawn.
*/
draw: function (context, tree) {
var config = {
maxDepth: 150,
levelSeparation: 50,
siblingSeparation: 40,
subtreeSeparation: 40,
topXAdjustment: 0,
topYAdjustment: 40
},
maxLevelHeight = [],
maxLevelWidth = [],
previousLevelTree = [],
rootXOffset = 0,
rootYOffset = 0,
/**
* Saves the height of a tree at a specified level.
* @param tree The tree.
* @param level The current vertical level of the tree.
*/
setLevelHeight = function (tree, level) {
maxLevelHeight[level] = tree.height;
},
/**
* Saves the width of a tree at a specified level.
* @param tree The tree.
* @param level The current vertical level of the tree.
*/
setLevelWidth = function (tree, level) {
maxLevelWidth[level] = tree.width;
},
/**
* Sets the neighbors of the tree.
* @param tree The specified tree
* @param level The vertical level of the tree.
*/
setNeighbors = function (tree, level) {
tree.leftNeighbor = previousLevelTree[level];
if (tree.leftNeighbor)
tree.leftNeighbor.rightNeighbor = tree;
previousLevelTree[level] = tree;
},
/**
* Returns the leftmost descendant of the tree.
* @param tree The specified tree.
* @param level The vertical level of the tree.
* @param maxlevel The maximum level in which to stop searching.
* @returns {*} The leftmost descendant if found, or null.
*/
getLeftMost = function (tree, level, maxlevel) {
if (level >= maxlevel) return tree;
if (tree.numChildren() === 0) return null;
var n = tree.numChildren();
for (var i = 0; i < n; i++) {
var iChild = tree.getChildAt(i);
var leftmostDescendant = getLeftMost(iChild, level + 1, maxlevel);
if (leftmostDescendant !== null)
return leftmostDescendant;
}
return null;
},
/**
* Gets the width of the tree.
* @param tree The specified tree.
* @returns {number} The width of the tree.
*/
getNodeSize = function (tree) {
return tree.width;
},
/**
* Part of the first traversal of the tree for positioning. Smaller subtrees that could float between
* two adjacent larger subtrees are evenly spaced out.
* @param tree
* @param level
*/
apportion = function (tree, level) {
var firstChild = tree.getFirstChild(),
firstChildLeftNeighbor = firstChild.leftNeighbor,
j = 1;
for (var k = config.maxDepth - level; firstChild != null && firstChildLeftNeighbor != null && j <= k;) {
var modifierSumRight = 0;
var modifierSumLeft = 0;
var rightAncestor = firstChild;
var leftAncestor = firstChildLeftNeighbor;
for (var l = 0; l < j; l++) {
rightAncestor = rightAncestor.parentTree;
leftAncestor = leftAncestor.parentTree;
modifierSumRight += rightAncestor.modifier;
modifierSumLeft += leftAncestor.modifier;
}
var totalGap = (firstChildLeftNeighbor.prelim + modifierSumLeft + getNodeSize(firstChildLeftNeighbor) + config.subtreeSeparation) - (firstChild.prelim + modifierSumRight);
if (totalGap > 0) {
var subtreeAux = tree;
var numSubtrees = 0;
for (; subtreeAux != null && subtreeAux != leftAncestor; subtreeAux = subtreeAux.getLeftSibling()) {
numSubtrees++;
}
if (subtreeAux != null) {
var subtreeMoveAux = tree;
var singleGap = totalGap / numSubtrees;
for (; subtreeMoveAux != leftAncestor; subtreeMoveAux = subtreeMoveAux.getLeftSibling()) {
subtreeMoveAux.prelim += totalGap;
subtreeMoveAux.modifier += totalGap;
totalGap -= singleGap;
}
}
}
j++;
if (firstChild.numChildren() == 0) {
firstChild = getLeftMost(tree, 0, j);
} else {
firstChild = firstChild.getFirstChild();
}
if (firstChild != null) {
firstChildLeftNeighbor = firstChild.leftNeighbor;
}
}
},
/**
* A postorder traversal of the tree. Each subtree is manipulated recursively from the bottom to top
* and left to right, positioning the rigid units that form each subtree until none are touching each
* other. Smaller subtrees are combined, forming larger subtrees until the root has been reached.
* @param tree
* @param level
*/
firstWalk = function (tree, level) {
var leftSibling = null;
tree.xPos = 0;
tree.yPos = 0;
tree.prelim = 0;
tree.modifier = 0;
tree.leftNeighbor = null;
tree.rightNeighbor = null;
setLevelHeight(tree, level);
setLevelWidth(tree, level);
setNeighbors(tree, level);
if (tree.numChildren() === 0 || level == config.maxDepth) {
leftSibling = tree.getLeftSibling();
if (leftSibling !== null)
tree.prelim = leftSibling.prelim + getNodeSize(leftSibling) + config.siblingSeparation;
else
tree.prelim = 0;
}
else {
var n = tree.numChildren();
for (var i = 0; i < n; i++) {
firstWalk(tree.getChildAt(i), level + 1);
}
var midPoint = tree.getChildrenCenter();
midPoint -= getNodeSize(tree) / 2;
leftSibling = tree.getLeftSibling();
if (leftSibling) {
tree.prelim = leftSibling.prelim + getNodeSize(leftSibling) + config.siblingSeparation;
tree.modifier = tree.prelim - midPoint;
apportion(tree, level);
}
else {
tree.prelim = midPoint;
}
}
},
/**
* A preorder traversal. Each node is given it's final X,Y coordinates by summing the preliminary
* coordinate and the modifiers of all of its ancestor trees.
* @param tree The tree that will be traversed.
* @param level The vertical level of the tree.
* @param X The X value of the tree.
* @param Y The Y value of the tree.
*/
secondWalk = function (tree, level, X, Y) {
tree.xPos = rootXOffset + tree.prelim + X;
tree.yPos = rootYOffset + Y;
if (tree.numChildren())
secondWalk(tree.getFirstChild(), level + 1, X + tree.modifier, Y + maxLevelHeight[level] + config.levelSeparation);
var rightSibling = tree.getRightSibling();
if (rightSibling)
secondWalk(rightSibling, level, X, Y);
},
/**
* Assign X,Y position values to the tree and it's descendants.
* @param tree The tree to be positioned.
*/
positionTree = function (tree) {
maxLevelHeight = [];
maxLevelWidth = [];
previousLevelTree = [];
firstWalk(tree, 0);
rootXOffset = config.topXAdjustment + tree.xPos;
rootYOffset = config.topYAdjustment + tree.yPos;
secondWalk(tree, 0, 0, 0);
rootXOffset = Math.abs(getMinX(tree)); //Align to left
secondWalk(tree, 0, 0, 0);
},
getMinX = function (tree) {
var nodes = TREE.getNodeList(tree);
var min = 0;
for (var i = 0; i < nodes.length; i++){
if (nodes[i].xPos < min)
min = nodes[i].xPos;
}
return min;
},
/**
* Draw the tree and it's descendants on the canvass.
* @param tree The tree that will be drawn.
*/
drawNode = function (tree) {
var x = tree.xPos,
y = tree.yPos,
width = tree.width,
height = tree.height,
text = tree.text,
textWidth = context.measureText(text).width;
context.beginPath();
context.moveTo(x, y);
context.lineTo(x, y + height);
context.lineTo(x + width, y + height);
context.lineTo(x + width, y);
context.lineTo(x, y);
context.lineWidth = 1;
context.fillStyle = tree.bgcolor;
context.fill();
context.stroke();
context.strokeStyle = tree.color;
context.textBaseline = 'middle';
context.strokeText(text, (x + width / 2) - textWidth / 2, y + height / 2, width);
context.strokeStyle = "black";
if (tree.children.length > 0) {
context.beginPath();
context.moveTo(x + width / 2, y + height);
context.lineTo(x + width / 2, y + height + config.levelSeparation / 2);
context.moveTo(tree.getFirstChild().xPos + tree.getFirstChild().width / 2, y + height + config.levelSeparation / 2);
context.lineTo(tree.getLastChild().xPos + tree.getLastChild().width / 2, y + height + config.levelSeparation / 2);
context.stroke();
}
if (tree.parentId != -1) {
context.beginPath();
context.moveTo(x + width / 2, y);
context.lineTo(x + width / 2, y - config.levelSeparation / 2);
context.stroke();
}
for (var i = 0; tree.numChildren() > 0 && i < tree.numChildren(); i++) {
drawNode(tree.getChildAt(i));
}
};
positionTree(tree);
this.clear(context);
drawNode(tree);
}
};
}());</script>
<script>//comment
var maintree;
function initialize() {
var array = <?= $JsonArray; ?>;
var superParent = $.grep(array, function(dt) {
return dt.parent === null;
});
var currNode = $.grep(array, function(dt) {
return dt.id === null;
});
var canvas = document.getElementById("canvas"),
context = canvas.getContext("2d"),
tree = TREE.create(superParent[0].name),
nodes = TREE.getNodeList(tree),
currNode = tree,
add_child_button = document.getElementById("add_child"),
remove_node = document.getElementById("remove_node"),
zoom_in = document.getElementById("zoom_in"),
zoom_out = document.getElementById("zoom_out");
canvas.addEventListener("click", function (event) {
var x = event.pageX - canvas.offsetLeft,
y = event.pageY - canvas.offsetTop;
for (var i = 0; i < nodes.length; i++) {
if (x > nodes[i].xPos && y > nodes[i].yPos && x < nodes[i].xPos + nodes[i].width && y < nodes[i].yPos + nodes[i].height) {
currNode.selected(false);
nodes[i].selected(true);
currNode = nodes[i];
TREE.clear(context);
TREE.draw(context, tree);
updatePage(currNode);
break;
}
}
}, false);
canvas.addEventListener("mousemove", function (event) {
var x = event.pageX - canvas.offsetLeft,
y = event.pageY - canvas.offsetTop;
for (var i = 0; i < nodes.length; i++) {
if (x > nodes[i].xPos && y > nodes[i].yPos && x < nodes[i].xPos + nodes[i].width && y < nodes[i].yPos + nodes[i].height) {
canvas.style.cursor = "pointer";
break;
}
else {
canvas.style.cursor = "auto";
}
}
}, false);
add_child_button.addEventListener('click', function (event)
{
var person = prompt("Please enter Subject name", "child of "+currNode);
currNode.addChild(TREE.create(person));
TREE.clear(context);
nodes = TREE.getNodeList(tree);
TREE.draw(context, tree,parent);
$.ajax({
type: "GET",
url: "test1.php",
data: data
}).done(function( event ) {
alert("Please enter Subject name", "child of "+currNode);
});
}, false)
;
remove_node.addEventListener('click', function (event) {
TREE.destroy(currNode);
TREE.clear(context);
nodes = TREE.getNodeList(tree);
TREE.draw(context, tree);
}, false);
zoom_in.addEventListener('click', function (event) {
for (var i = 0; i < nodes.length; i++){
nodes[i].width *= 1.05;
nodes[i].height *= 1.05;
}
TREE.config.width *= 1.05;
TREE.config.height *= 1.05;
TREE.clear(context);
TREE.draw(context, tree);
}, false);
zoom_out.addEventListener('click', function (event) {
for (var i = 0; i < nodes.length; i++){
nodes[i].width = nodes[i].width * 0.95;
nodes[i].height = nodes[i].height * 0.95;
}
TREE.config.width *= 0.95;
TREE.config.height *= 0.95;
TREE.clear(context);
TREE.draw(context, tree);
}, false);
/* GO.addEventListener('click', function (event) {
var el = document.getElementById('GO');
el.onclick = showFoo;
function showFoo() {
alert('I am foo!');
return false;
},false);
<a href="f.html" title="Get some foo!" id="foo">Show me some foo</a>*/
context.canvas.width = document.getElementById("main").offsetWidth;
context.canvas.height = document.getElementById("main").offsetHeight;
populateDummyData(tree);
nodes = TREE.getNodeList(tree);
TREE.draw(context, tree);
maintree = tree;
}
function updatePage(tree) {
var info_panel = document.getElementById("information_panel").innerHTML = location.href;;
var header = document.getElementById("header");
header.innerHTML = "CIS Family";
var info_panel_html = "<ul>";
info_panel_html += "<li> Name of perant: " +tree.text +"</li>";
info_panel_html += "<li> summary: " +"c++" +"</li>";
info_panel_html += "</ul>";
info_panel.innerHTML = info_panel_html;
}
function myFunction() {
document.getElementById("myAnchor").href = "http://www.cnn.com/";
document.getElementById("demo").innerHTML = "The link above now goes to www.cnn.com.";
}
function populateDummyData(tree, parent) {
tree.selected(true);
updatePage(tree);
parent = "1";
var array = <?= $JsonArray; ?>;
var children = $.grep(array, function(dt) {
return dt.parent === parent;
});
for (var i = 0; i < children.length; i++) {
tree.addChild(TREE.create(children[i].name));
}
parent = "2";
var array = <?= $JsonArray; ?>;
var children = $.grep(array, function(dt) {
return dt.parent === parent;
});
for (var i = 0; i < children.length; i++) {
tree.getDescendent(2).addChild(TREE.create(children[i].name));
}
parent = "3";
var array = <?= $JsonArray; ?>;
var children = $.grep(array, function(dt) {
return dt.parent === parent;
});
for (var i = 0; i < children.length; i++) {
tree.getDescendent(3).addChild(TREE.create(children[i].name));
}
parent = "4";
var array = <?= $JsonArray; ?>;
var children = $.grep(array, function(dt) {
return dt.parent === parent;
});
for (var i = 0; i < children.length; i++) {
tree.getDescendent(4).addChild(TREE.create(children[i].name));
}
parent = "5";
var array = <?= $JsonArray; ?>;
var children = $.grep(array, function(dt) {
return dt.parent === parent;
});
for (var i = 0; i < children.length; i++) {
tree.getDescendent(5).addChild(TREE.create(children[i].name));
}
parent = "6";
var array = <?= $JsonArray; ?>;
var children = $.grep(array, function(dt) {
return dt.parent === parent;
});
for (var i = 0; i < children.length; i++) {
tree.getDescendent(6).addChild(TREE.create(children[i].name));
}
parent = "7";
var array = <?= $JsonArray; ?>;
var children = $.grep(array, function(dt) {
return dt.parent === parent;
});
for (var i = 0; i < children.length; i++) {
tree.getDescendent(7).addChild(TREE.create(children[i].name));
}
/* tree.addChild(TREE.create("logic"));
tree.addChild(TREE.create("c#"));
tree.getChildAt(0).addChild(TREE.create("network"));
tree.getChildAt(1).addChild(TREE.create("data base");
tree.getChildAt(1).addChild(TREE.create("java"));
tree.getChildAt(1).addChild(TREE.create("data structure"));
tree.getDescendent(4).addChild(TREE.create("data control"));
tree.getDescendent(4).addChild(TREE.create("wirless"));
tree.getDescendent(4).addChild(TREE.create("ds"));
tree.getDescendent(5).addChild(TREE.create("anlysis"));
tree.getDescendent(5).addChild(TREE.create("DBMS"));
tree.getDescendent(5).addChild(TREE.create("IR"));
tree.getDescendent(5).addChild(TREE.create("data base 2"));
tree.getDescendent(6).addChild(TREE.create("web"));
tree.getDescendent(6).addChild(TREE.create("vb"));
tree.getDescendent(7).addChild(TREE.create("algorthim"));
tree.getDescendent(7).addChild(TREE.create("data structure 2"));
tree.getDescendent(7).addChild(TREE.create("OS"));
tree.getDescendent(11).addChild(TREE.create("software engineer"));
tree.getDescendent(12).addChild(TREE.create("Data warehousing"));
tree.getDescendent(13).addChild(TREE.create("Data minig"));
tree.getDescendent(13).addChild(TREE.create("GIS"));
tree.getDescendent(14).addChild(TREE.create("database2"));
tree.getDescendent(15).addChild(TREE.create("css"));
tree.getDescendent(15).addChild(TREE.create(""));
tree.getDescendent(15).addChild(TREE.create("topics cis"));
tree.getDescendent(16).addChild(TREE.create("image processing"));
tree.getDescendent(16).addChild(TREE.create("multi media"));
tree.getDescendent(17).addChild(TREE.create("AI"));
tree.getDescendent(17).addChild(TREE.create("data structure2"));
tree.getDescendent(19).addChild(TREE.create("computer arachclucter"));
*/
}</script>
<div style=" position: absolute;
height: 170px;
top: 750px;
left:80px;
width: 200px;
padding: 10px;
border: 2px solid black;
margin: 0;">
<div style="margin-left: 30px;margin-top: 25px;">
<div style="color:#a9bbc5;text-align:left; background-color: #4a5662; width: 80px;
border: 5px solid black;
padding: 15px;
"><b>Arbic 1</b></div>
<div style="color:#a9bbc5;text-align:left;background-color: #4a5662; width: 80px;
border: 5px solid black;
padding: 15px;
"><b>Arbic 2</b></div> </div>
</div>
<div style=" position: absolute; margin-left: 100px;
height: 170px;
top: 750px;
left:303px;
width: 200px;
padding: 10px;
border: 2px solid black;
margin: 0;"> <div style="margin-left: 30px; margin-top: 25px;
">
<div style="color:#a9bbc5;text-align:left; background-color:#4a5662; width: 80px;
border: 5px solid black;
padding: 15px;
"><b>English 1</b></div>
<div style="color:#a9bbc5;text-align:left;background-color:#4a5662; width: 80px;
border: 5px solid black;
padding: 15px;
"><b>English 2</b></div> </div>
</div>
<div style=" position: absolute;
height: 170px;
top: 750px;
left:524px;
width: 200px;
padding: 10px;
border: 2px solid black;
margin: 0;">
<div style="margin-left: 10px;margin-top: 25px;">
<div style="color:#a9bbc5;text-align:left; background-color:#4a5662; width: 145px;
border: 5px solid black;
padding: 15px;
"><b>National Education</b></div>
<div style="color:#a9bbc5;text-align:left;background-color:#4a5662; width: 145px;
border: 5px solid black;
padding: 15px;
"><b>Military Science</b></div> </div>
</div>
<div style=" position: absolute; margin-left: 100px;
height: 170px;
top: 750px;
left:800px;
width: 200px;
padding: 10px;
border: 2px solid black;
margin: 0;"> <div style="margin-left: 30px; margin-top: 0px;
">
<div style="color:#a9bbc5;text-align:left; background-color:#4a5662; width: 110px;
border: 5px solid black;
padding: 15px;
"> <b>calculus 1</b></div>
<div style="color:black;text-align:left;background-color:#4a5662; width: 110px;
border: 5px solid black;
padding: 15px;
"><b>calculus 2</b></div> <div style="color:#a9bbc5;text-align:left;background-color:#4a5662; width: 110px;
border: 5px solid black;
padding: 15px;
"><b>physics for IT</b></div> </div>
</div>
<h2 style="margin-left: 250px;margin-top: 670px;position: absolute; text-align: justify;
"><b>University Requirement</b>
</h2><h2 style="margin-left: 780px;margin-top: 670px;position: absolute; text-align: justify;
"><b>College Requirement</b>
</h2>
</body>
</html>
ساعد بالإجابة
"إن في قضاء حوائج الناس لذة لا يَعرفها إلا من جربها، فافعل الخير مهما استصغرته فإنك لا تدري أي حسنة تدخلك الجنة."
الإجابات (1)
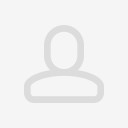
السؤال والكود غير معروفين
يرجى تحديد ماهو السؤال ووضع الأكواد في المحرر الخاص بها الموجود مع المحرر
حتى يتم الاجابة على سؤالك
لايوجد لديك حساب في عالم البرمجة؟
تحب تنضم لعالم البرمجة؟ وتنشئ عالمك الخاص، تنشر المقالات، الدورات، تشارك المبرمجين وتساعد الآخرين، اشترك الآن بخطوات يسيرة !