احتاج مساعده في تعديل كود c++
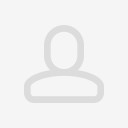
اهلًا يا اصحاب هذا الكود search and Remove__End ما تشتغل وأيضا احتاج أضيف حذف من البدايه وأضافه من النهايه
/*
* C++ Program to Implement Doubly Linked List
*/
#include<iostream>
#include<cstdio>
#include<cstdlib>
/*
* Node Declaration
*/
using namespace std;
struct node
{
int info;
struct node *next;
struct node *prev;
}*start;
/*
Class Declaration
*/
class double_llist
{
public:
void create_list(int value);
void Insert_beginning(int value);
void Insert_Afterx(int value, int position);
void Insert_End(int value);
void Remove_Afterx(int value);
void search_element(int value);
void display_dlist();
void search();
double_llist()
{
start = NULL;
}
};
/*
* Main: Conatins Menu
*/
int main()
{
int choice, element, position,i=0,flag;
double_llist dl ;
while (1)
{
cout<<endl<<"----------------------------"<<endl;
cout<<endl<<"Operations on Doubly linked list"<<endl;
cout<<endl<<"----------------------------"<<endl;
cout<<"1.Create Node:"<<endl;
cout<<"2.Insert the item at begining:"<<endl;
cout<<"3.Insert the item after value X:"<<endl;
cout<<"4.isert at end"<<endl;
cout<<"5.Remove the item after valueX:"<<endl;
cout<<"6.Display"<<endl;
cout<<"7. exit"<<endl;
cout<<"8.search"<<endl;
cout<<"Enter your choice : ";
cin>>choice;
switch ( choice )
{
case 1:
cout<<"Enter the element: ";
cin>>element;
dl.create_list(element);
cout<<endl;
break;
case 2:
cout<<"Enter the element: ";
cin>>element;
dl.Insert_beginning(element);
cout<<endl;
break;
case 3:
cout<<"Enter the element: ";
cin>>element;
cout<<"Insert Element after postion: ";
cin>>position;
dl.Insert_Afterx(element, position);
cout<<endl;
break;
case 4:
cout<<"Enter the element: ";
cin>>element;
dl.Insert_End(element);
cout<<endl;
break;
case 5:
if (start == NULL)
{
cout<<"List empty,nothing to delete"<<endl;
break;
}
cout<<"Enter the element for deletion: ";
cin>>element;
dl.Remove_Afterx(element);
cout<<endl;
break;
case 6:
dl.display_dlist();
cout<<endl;
break;
case 7:
exit(1);
default:
cout<<"Wrong choice"<<endl;
case 8:
if (start == NULL)
{
cout<<"List empty,nothing to search"<<endl;
break;
}
dl.search();
break;
}
}
return 0;
}
/*
* Create Double Link List
*/
void double_llist::create_list(int value)
{
struct node *s, *temp;
temp = new(struct node);
temp->info = value;
temp->next = NULL;
if (start == NULL)
{
temp->prev = NULL;
start = temp;
}
else
{
s = start;
while (s->next != NULL)
s = s->next;
s->next = temp;
temp->prev = s;
}
}
/*
* Insertion at the beginning
*/
void double_llist::Insert_beginning(int value)
{
if (start == NULL)
{
cout<<"First Create the list."<<endl;
return;
}
struct node *temp;
temp = new(struct node);
temp->prev = NULL;
temp->info = value;
temp->next = start;
start->prev = temp;
start = temp;
cout<<"Element Inserted"<<endl;
}
void double_llist::Insert_End(int value)
{ if (start == NULL)
{
cout<<"First Create the list."<<endl;
return;
}
struct node *tmp, *q;
int i;
q = start;
tmp = new(struct node);
tmp->info = value;
if (q->next == NULL)
{ tmp->next = q->next;
tmp->next->prev = tmp;
q->next = tmp;
tmp->prev = q;
}
}
/*
* Insertion of element at a particular position
*/
void double_llist::Insert_Afterx(int value, int pos)
{
if (start == NULL)
{
cout<<"First Create the list."<<endl;
return;
}
struct node *tmp, *q;
int i;
q = start;
for (i = 0;i < pos - 1;i++)
{
q = q->next;
if (q == NULL)
{
cout<<"There are less than ";
cout<<pos<<" elements."<<endl;
return;
}
}
tmp = new(struct node);
tmp->info = value;
if (q->next == NULL)
{
q->next = tmp;
tmp->next = NULL;
tmp->prev = q;
}
else
{
tmp->next = q->next;
tmp->next->prev = tmp;
q->next = tmp;
tmp->prev = q;
}
cout<<"Element Inserted"<<endl;
}
/*
* Deletion of element from the list
*/
void double_llist::Remove_Afterx(int value)
{
struct node *tmp, *q;
/*first element deletion*/
if (start->info == value)
{
tmp = start;
start = start->next;
start->prev = NULL;
cout<<"Element Deleted"<<endl;
free(tmp);
return;
}
q = start;
while (q->next->next != NULL)
{
/*Element deleted in between*/
if (q->next->info == value)
{
tmp = q->next;
q->next = tmp->next;
tmp->next->prev = q;
cout<<"Element Deleted"<<endl;
free(tmp);
return;
}
q = q->next;
}
/*last element deleted*/
if (q->next->info == value)
{
tmp = q->next;
free(tmp);
q->next = NULL;
cout<<"Element Deleted"<<endl;
return;
}
cout<<"Element "<<value<<" not found"<<endl;
}
/*
* Display elements of Doubly Link List
*/
void double_llist::display_dlist()
{
struct node *q;
if (start == NULL)
{
cout<<"List empty,nothing to display"<<endl;
return;
}
q = start;
cout<<"The Doubly Link List is :"<<endl;
while (q != NULL)
{
cout<<q->info<<" <-> ";
q = q->next;
}
cout<<"NULL"<<endl;
}
void double_llist:: search()
{
struct node *ptr;
int item,i=0,flag;
struct node *head;
ptr = head;
if(ptr == NULL)
{
cout<<"\nEmpty List\n";
}
else
{
cout<<"\nEnter item which you want to search?\n";
cin>>item;
while (ptr!=NULL)
{
if(ptr->info == item)
{
cout<<"\nitem found at location %d ",i+1;
flag=0;
break;
}
else
{
flag=1;
}
i++;
ptr = ptr -> next;
}
if(flag==1)
{
cout<<"\nItem not found\n";
}
}
}تم
ساعد بالإجابة
"إن في قضاء حوائج الناس لذة لا يَعرفها إلا من جربها، فافعل الخير مهما استصغرته فإنك لا تدري أي حسنة تدخلك الجنة."
الإجابات (1)
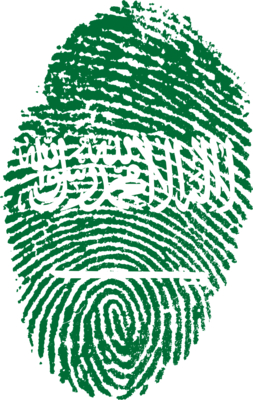
هلا اخوي, الكود شغال مافيه شي,
هذا ال output, والكود يليه:
الكود:
/*
* C++ Program to Implement Doubly Linked List
*/
#include<iostream>
#include<cstdio>
#include<cstdlib>
/*
* Node Declaration
*/
using namespace std;
struct node
{
int info;
struct node * next;
struct node * prev;
}* start;
/*
Class Declaration
*/
class double_llist
{
public:
void create_list(int value);
void Insert_beginning(int value);
void Insert_Afterx(int value, int position);
void Insert_End(int value);
void Remove_Afterx(int value);
void search_element(int value);
void display_dlist();
void search();
double_llist()
{
start = NULL;
}
};
/*
* Main: Conatins Menu
*/
int main()
{
int choice, element, position, i = 0, flag;
double_llist dl;
while (1)
{
cout << endl << "----------------------------" << endl;
cout << endl << "Operations on Doubly linked list" << endl;
cout << endl << "----------------------------" << endl;
cout << "1.Create Node:" << endl;
cout << "2.Insert the item at begining:" << endl;
cout << "3.Insert the item after value X:" << endl;
cout << "4.isert at end" << endl;
cout << "5.Remove the item after valueX:" << endl;
cout << "6.Display" << endl;
cout << "7. exit" << endl;
cout << "8.search" << endl;
cout << "Enter your choice : ";
cin >> choice;
switch (choice)
{
case 1:
cout << "Enter the element: ";
cin >> element;
dl.create_list(element);
cout << endl;
break;
case 2:
cout << "Enter the element: ";
cin >> element;
dl.Insert_beginning(element);
cout << endl;
break;
case 3:
cout << "Enter the element: ";
cin >> element;
cout << "Insert Element after postion: ";
cin >> position;
dl.Insert_Afterx(element, position);
cout << endl;
break;
case 4:
cout << "Enter the element: ";
cin >> element;
dl.Insert_End(element);
cout << endl;
break;
case 5:
if (start == NULL)
{
cout << "List empty,nothing to delete" << endl;
break;
}
cout << "Enter the element for deletion: ";
cin >> element;
dl.Remove_Afterx(element);
cout << endl;
break;
case 6:
dl.display_dlist();
cout << endl;
break;
case 7:
exit(1);
default:
cout << "Wrong choice" << endl;
case 8:
if (start == NULL)
{
cout << "List empty,nothing to search" << endl;
break;
}
dl.search();
break;
}
}
return 0;
}
/*
* Create Double Link List
*/
void double_llist::create_list(int value)
{
struct node * s, * temp;
temp = new(struct node);
temp -> info = value;
temp -> next = NULL;
if (start == NULL)
{
temp -> prev = NULL;
start = temp;
} else
{
s = start;
while (s -> next != NULL)
s = s -> next;
s -> next = temp;
temp -> prev = s;
}
}
/*
* Insertion at the beginning
*/
void double_llist::Insert_beginning(int value)
{
if (start == NULL)
{
cout << "First Create the list." << endl;
return;
}
struct node * temp;
temp = new(struct node);
temp -> prev = NULL;
temp -> info = value;
temp -> next = start;
start -> prev = temp;
start = temp;
cout << "Element Inserted" << endl;
}
void double_llist::Insert_End(int value)
{
if (start == NULL)
{
cout << "First Create the list." << endl;
return;
}
struct node * tmp, * q;
int i;
q = start;
tmp = new(struct node);
tmp -> info = value;
if (q -> next == NULL)
{
tmp -> next = q -> next;
tmp -> next -> prev = tmp;
q -> next = tmp;
tmp -> prev = q;
}
}
/*
* Insertion of element at a particular position
*/
void double_llist::Insert_Afterx(int value, int pos)
{
if (start == NULL)
{
cout << "First Create the list." << endl;
return;
}
struct node * tmp, * q;
int i;
q = start;
for (i = 0; i < pos - 1; i++)
{
q = q -> next;
if (q == NULL)
{
cout << "There are less than ";
cout << pos << " elements." << endl;
return;
}
}
tmp = new(struct node);
tmp -> info = value;
if (q -> next == NULL)
{
q -> next = tmp;
tmp -> next = NULL;
tmp -> prev = q;
} else
{
tmp -> next = q -> next;
tmp -> next -> prev = tmp;
q -> next = tmp;
tmp -> prev = q;
}
cout << "Element Inserted" << endl;
}
/*
* Deletion of element from the list
*/
void double_llist::Remove_Afterx(int value)
{
struct node * tmp, * q;
/*first element deletion*/
if (start -> info == value)
{
tmp = start;
start = start -> next;
start -> prev = NULL;
cout << "Element Deleted" << endl;
free(tmp);
return;
}
q = start;
while (q -> next -> next != NULL)
{
/*Element deleted in between*/
if (q -> next -> info == value)
{
tmp = q -> next;
q -> next = tmp -> next;
tmp -> next -> prev = q;
cout << "Element Deleted" << endl;
free(tmp);
return;
}
q = q -> next;
}
/*last element deleted*/
if (q -> next -> info == value)
{
tmp = q -> next;
free(tmp);
q -> next = NULL;
cout << "Element Deleted" << endl;
return;
}
cout << "Element " << value << " not found" << endl;
}
/*
* Display elements of Doubly Link List
*/
void double_llist::display_dlist()
{
struct node * q;
if (start == NULL)
{
cout << "List empty,nothing to display" << endl;
return;
}
q = start;
cout << "The Doubly Link List is :" << endl;
while (q != NULL)
{
cout << q -> info << " <-> ";
q = q -> next;
}
cout << "NULL" << endl;
}
void double_llist::search()
{
struct node * ptr;
int item, i = 0, flag;
struct node * head;
ptr = head;
if (ptr == NULL)
{
cout << "\nEmpty List\n";
} else
{
cout << "\nEnter item which you want to search?\n";
cin >> item;
while (ptr != NULL)
{
if (ptr -> info == item)
{
cout << "\nitem found at location %d ", i + 1;
flag = 0;
break;
} else
{
flag = 1;
}
i++;
ptr = ptr -> next;
}
if (flag == 1)
{
cout << "\nItem not found\n";
}
}
}
لايوجد لديك حساب في عالم البرمجة؟
تحب تنضم لعالم البرمجة؟ وتنشئ عالمك الخاص، تنشر المقالات، الدورات، تشارك المبرمجين وتساعد الآخرين، اشترك الآن بخطوات يسيرة !