المكان المناسب لإنشاء واجهة مستخدم.
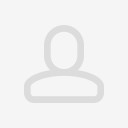
السلام عليكم ورحمة الله وبركاته
عندي الكود التالي ومطلوب مني إنشاء واجهة مستخدم له أبغى أستفسر عن الكلاس المناسب فيه لإنشاء الواجهة، أم هل يجب إنشاء كلاس أخرى خاصة بالواجهة؟وإذا كان لابد كيف أربطها بالكود؟
ملاحظة: الواجهةلابد تمثل الفعل الجاري حاليا من خلال الصور.
/**
* AlgorithmFactory.java
*
* This program tests Petersons solution to the critical section problem.
*
*/
public class AlgorithmFactory
{
public static void main(String args[]) {
MutualExclusion algorithm = new Petersons();
Thread first = new Thread(new Worker("Worker 0", 0, algorithm));
Thread second = new Thread(new Worker("Worker 1", 1, algorithm));
first.start();
second.start();
}
}
/**
* Petersons.java
*
* This program implements strict alternation as a means of handling synchronization.
*
* Note - Using an array for the two flag variables would be preferable, however we must
* declare the data as volatile and volatile does not extend to arrays.
*/
public class Petersons implements MutualExclusion
{
private volatile int turn;
private volatile boolean flag0;
private volatile boolean flag1;
public Petersons() {
flag0 = false;
flag1 = false;
turn = 0;
}
public void entrySection(int t) {
int other = 1 - t;
if (t == 0) {
turn = other;
flag0 = true;
while ( (flag1 == true) && (turn == other) )
Thread.yield();
}
else {
turn = other;
flag1 = true;
while ( (flag0 == true) && (turn == other) )
Thread.yield();
}
}
public void exitSection(int t) {
if(t == 0)
flag0 = false;
else
flag1 = false;
}
}
/**
* Worker.java
*
* This is a thread that is used to demonstrate solutions
* to the critical section problem.
*/
public class Worker implements Runnable
{
private String name;
private int id;
private MutualExclusion mutex;
public Worker(String name, int id, MutualExclusion mutex) {
this.name = name;
this.id = id;
this.mutex = mutex;
}
public void run() {
while (true) {
mutex.entrySection(id);
MutualExclusionUtilities.criticalSection(name);
mutex.exitSection(id);
MutualExclusionUtilities.nonCriticalSection(name);
}
}
}
/**
* MutualExclusion.java
*
* This interface is to be implmented by each solution to the critical section
* problem.
*/
public interface MutualExclusion {
public void entrySection(int turn);
public void exitSection(int turn);
}
/**
* MutualExclusionUtilities.java
*
* Utilities for simulating critical and non-critical sections.
*
*/
public class MutualExclusionUtilities
{
/**
* critical and non-critical sections are simulated by sleeping
* for a random amount of time between 0 and 3 seconds.
*/
public static void criticalSection(String name) {
System.out.println(name + " in critical section");
SleepUtilities.nap(3);
}
public static void nonCriticalSection(String name) {
System.out.println(name + " out of critical section");
SleepUtilities.nap(3);
}
}
/**
* utilities for causing a thread to sleep.
* Note, we should be handling interrupted exceptions
* but choose not to do so for code clarity.
*/
public class SleepUtilities
{
/**
* Nap between zero and NAP_TIME seconds.
*/
public static void nap() {
nap(NAP_TIME);
}
/**
* Nap between zero and duration seconds.
*/
public static void nap(int duration) {
int sleeptime = (int) (NAP_TIME * Math.random() );
try { Thread.sleep(sleeptime*1000); }
catch (InterruptedException e) {}
}
private static final int NAP_TIME = 5;
}
ساعد بالإجابة
"إن في قضاء حوائج الناس لذة لا يَعرفها إلا من جربها، فافعل الخير مهما استصغرته فإنك لا تدري أي حسنة تدخلك الجنة."
الإجابات (1)
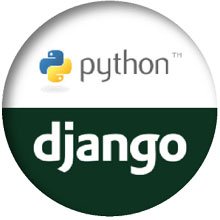
لن اجيب على السؤال هنا مختصين اكثر مني للاجابة على السؤال نفسه
لكن لو رغبت بالحصول على الاجابة بشكل افضل عليك بتنسيق الكود من خيارات الصندوق واختيار اضافة كود واختيار اللغة البرمجية المطلوبة
كالتالي
/**
* AlgorithmFactory.java
*
* This program tests Petersons solution to the critical section problem.
*
*/
public class AlgorithmFactory
{
public static void main(String args[]) {
MutualExclusion algorithm = new Petersons();
Thread first = new Thread(new Worker("Worker 0", 0, algorithm));
Thread second = new Thread(new Worker("Worker 1", 1, algorithm));
first.start();
second.start();
}
}
/**
* Petersons.java
*
* This program implements strict alternation as a means of handling synchronization.
*
* Note - Using an array for the two flag variables would be preferable, however we must
* declare the data as volatile and volatile does not extend to arrays.
*/
public class Petersons implements MutualExclusion
{
private volatile int turn;
private volatile boolean flag0;
private volatile boolean flag1;
public Petersons() {
flag0 = false;
flag1 = false;
turn = 0;
}
public void entrySection(int t) {
int other = 1 - t;
if (t == 0) {
turn = other;
flag0 = true;
while ( (flag1 == true) && (turn == other) )
Thread.yield();
}
else {
turn = other;
flag1 = true;
while ( (flag0 == true) && (turn == other) )
Thread.yield();
}
}
public void exitSection(int t) {
if(t == 0)
flag0 = false;
else
flag1 = false;
}
}
/**
* Worker.java
*
* This is a thread that is used to demonstrate solutions
* to the critical section problem.
*/
public class Worker implements Runnable
{
private String name;
private int id;
private MutualExclusion mutex;
public Worker(String name, int id, MutualExclusion mutex) {
this.name = name;
this.id = id;
this.mutex = mutex;
}
public void run() {
while (true) {
mutex.entrySection(id);
MutualExclusionUtilities.criticalSection(name);
mutex.exitSection(id);
MutualExclusionUtilities.nonCriticalSection(name);
}
}
}
/**
* MutualExclusion.java
*
* This interface is to be implmented by each solution to the critical section
* problem.
*/
public interface MutualExclusion {
public void entrySection(int turn);
public void exitSection(int turn);
}
/**
* MutualExclusionUtilities.java
*
* Utilities for simulating critical and non-critical sections.
*
*/
public class MutualExclusionUtilities
{
/**
* critical and non-critical sections are simulated by sleeping
* for a random amount of time between 0 and 3 seconds.
*/
public static void criticalSection(String name) {
System.out.println(name + " in critical section");
SleepUtilities.nap(3);
}
public static void nonCriticalSection(String name) {
System.out.println(name + " out of critical section");
SleepUtilities.nap(3);
}
}
/**
* utilities for causing a thread to sleep.
* Note, we should be handling interrupted exceptions
* but choose not to do so for code clarity.
*/
public class SleepUtilities
{
/**
* Nap between zero and NAP_TIME seconds.
*/
public static void nap() {
nap(NAP_TIME);
}
/**
* Nap between zero and duration seconds.
*/
public static void nap(int duration) {
int sleeptime = (int) (NAP_TIME * Math.random() );
try { Thread.sleep(sleeptime*1000); }
catch (InterruptedException e) {}
}
private static final int NAP_TIME = 5;
}
لايوجد لديك حساب في عالم البرمجة؟
تحب تنضم لعالم البرمجة؟ وتنشئ عالمك الخاص، تنشر المقالات، الدورات، تشارك المبرمجين وتساعد الآخرين، اشترك الآن بخطوات يسيرة !